Turbo Native Modules: iOS
Now it's time to write some iOS platform code to make sure localStorage
survives after the application is closed.
Prepare your Xcode Project
We need to prepare your iOS project using Xcode. After completing these 6 steps you'll have RCTNativeLocalStorage
that implements the generated NativeLocalStorageSpec
interface.
- Open the CocoPods generated Xcode Workspace:
cd ios
open TurboModuleExample.xcworkspace
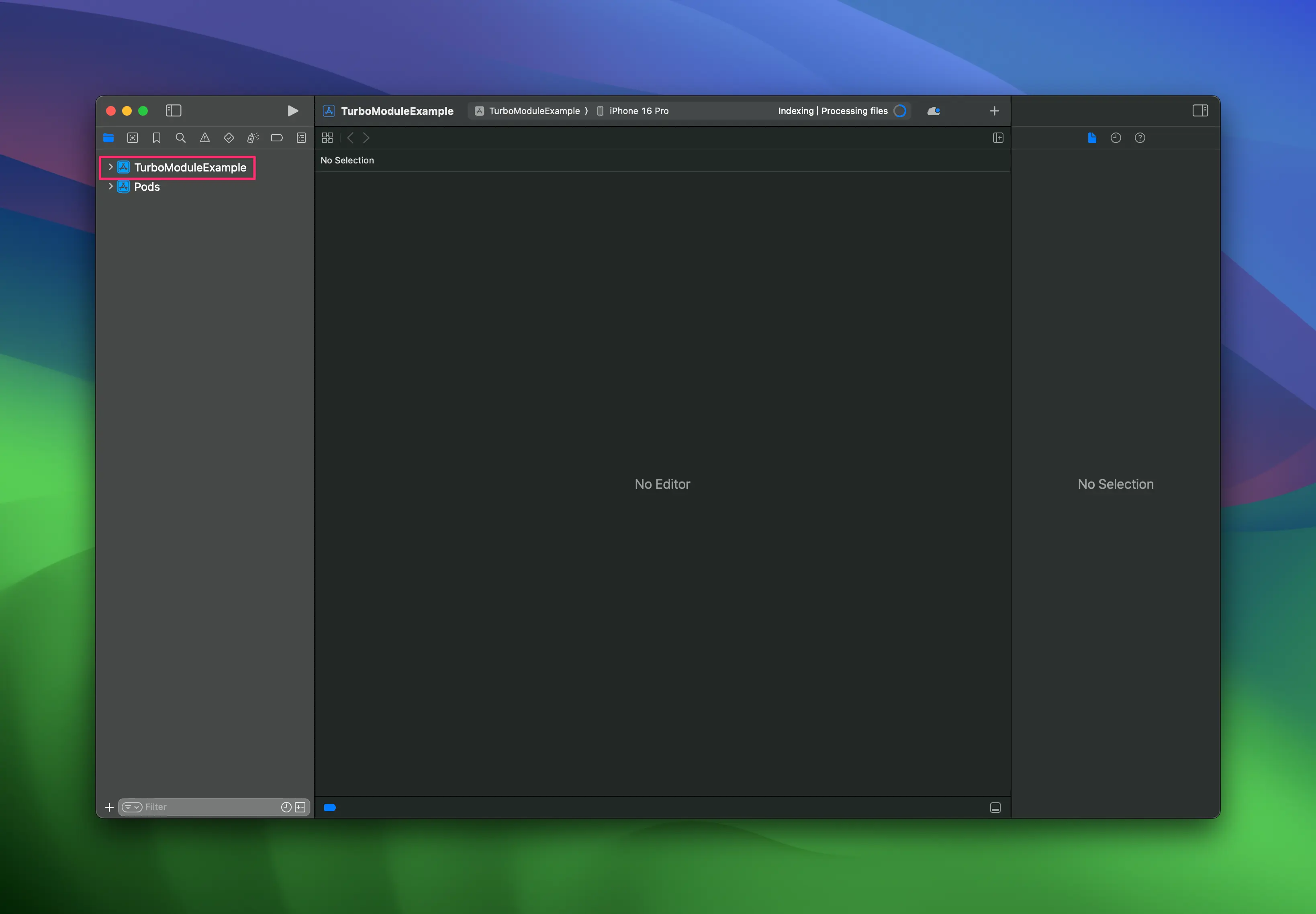
- Right click on app and select
New Group
, call the new groupNativeLocalStorage
.
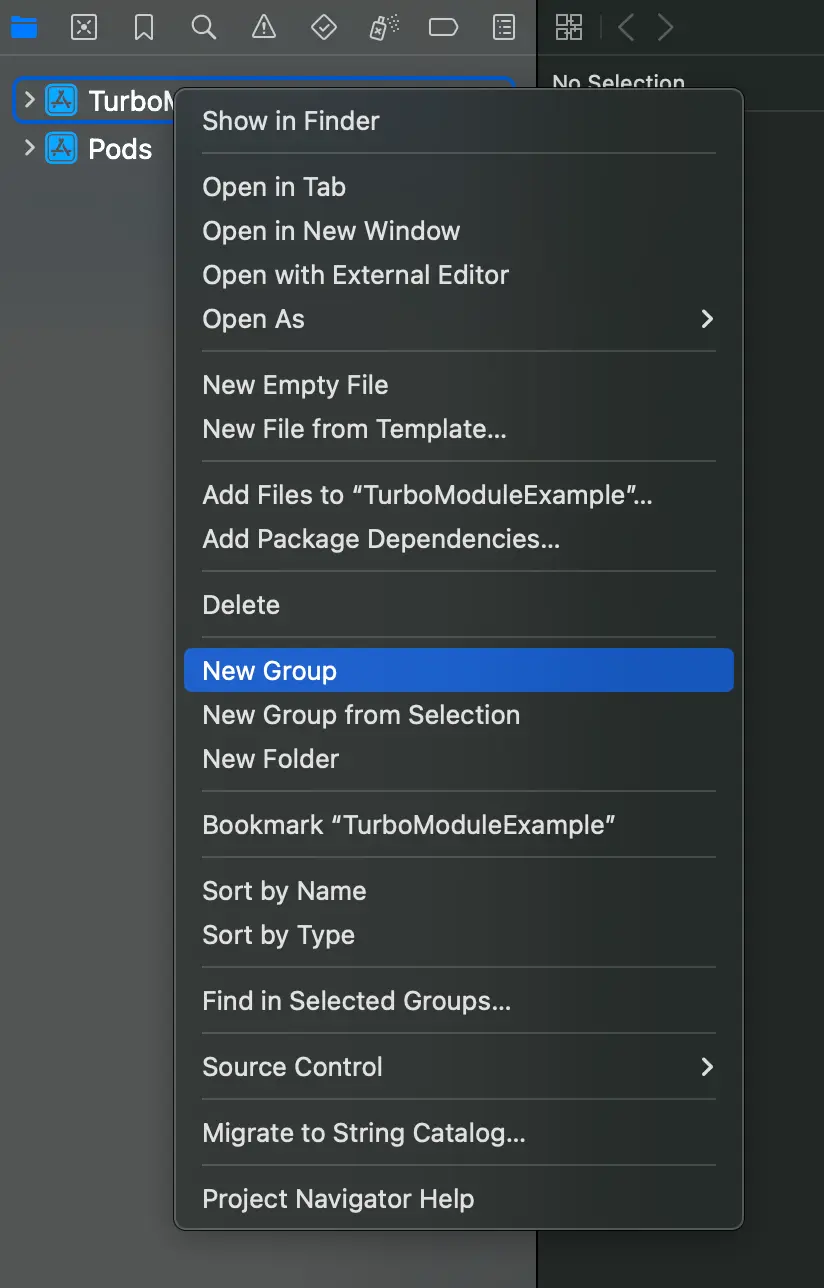
- In the
NativeLocalStorage
group, createNew
→File from Template
.
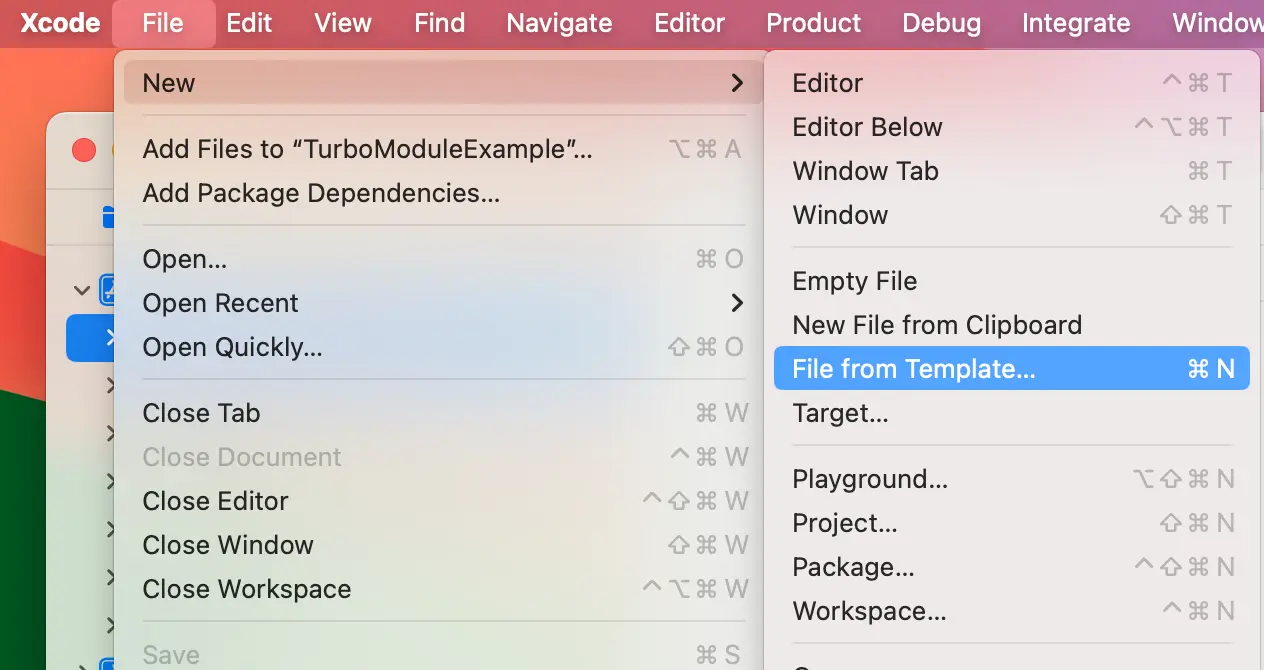
- Use the
Cocoa Touch Class
.
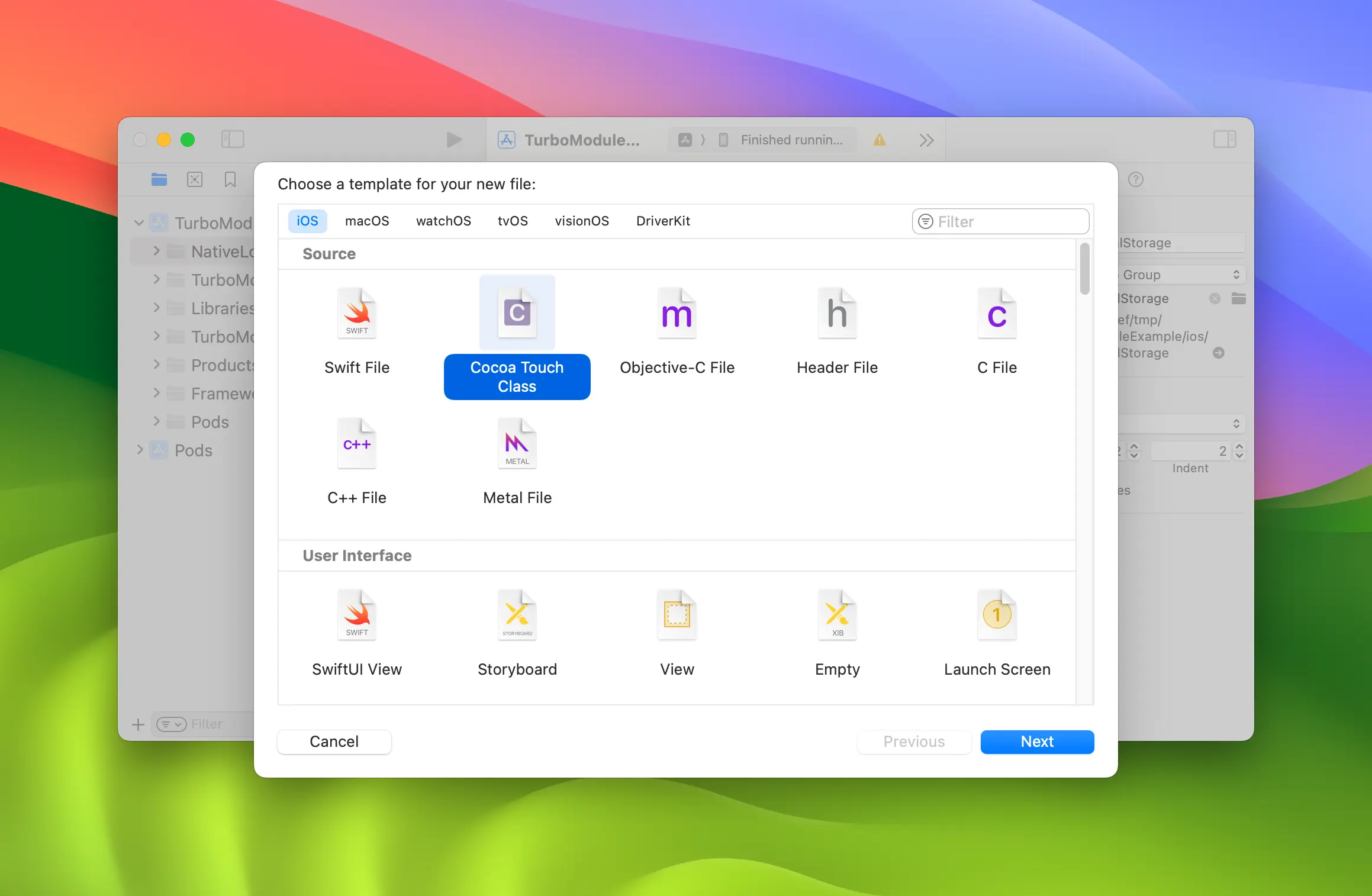
- Name the Cocoa Touch Class
RCTNativeLocalStorage
with theObjective-C
language.
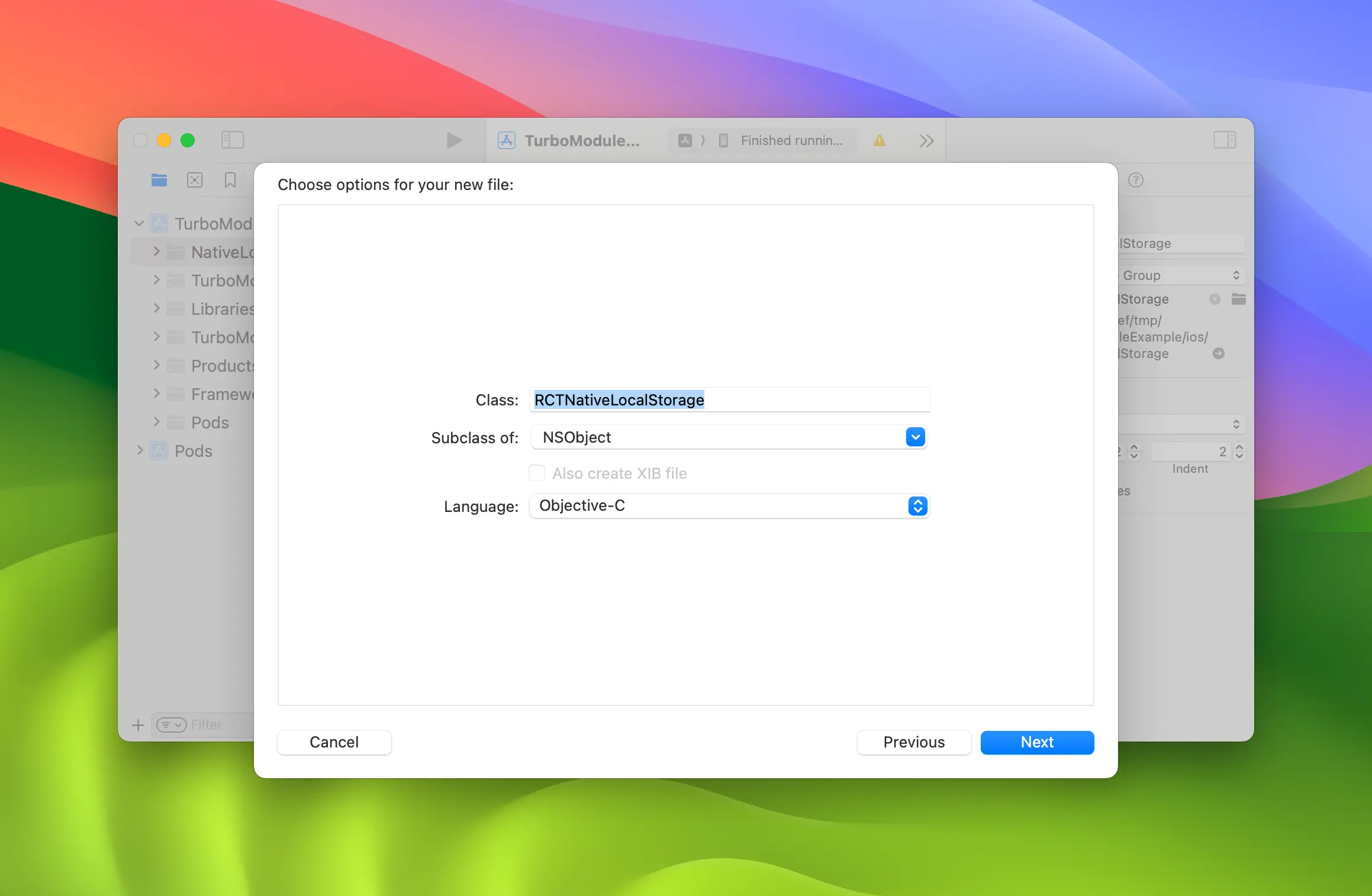
- Rename
RCTNativeLocalStorage.m
→RCTNativeLocalStorage.mm
making it an Objective-C++ file.
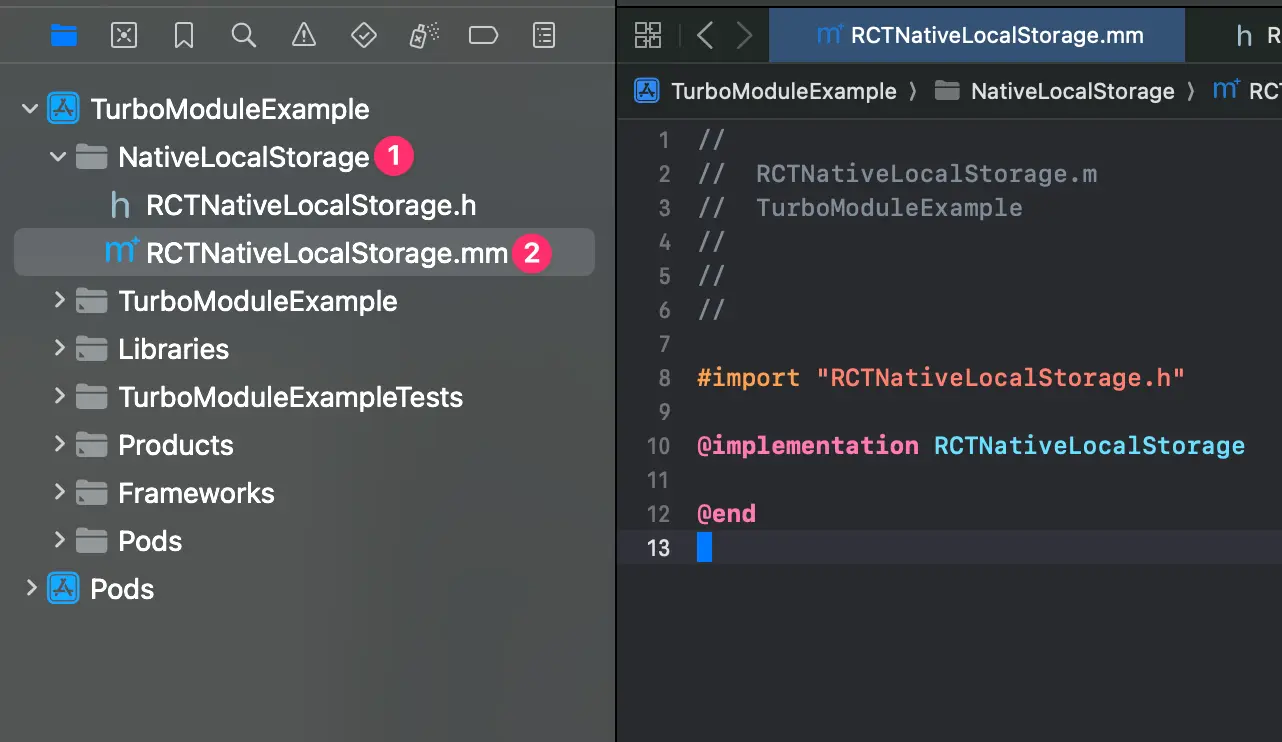
Implement localStorage with NSUserDefaults
Start by updating RCTNativeLocalStorage.h
:
// RCTNativeLocalStorage.h
// TurboModuleExample
#import <Foundation/Foundation.h>
#import <NativeLocalStorageSpec/NativeLocalStorageSpec.h>
NS_ASSUME_NONNULL_BEGIN
@interface RCTNativeLocalStorage : NSObject
@interface RCTNativeLocalStorage : NSObject <NativeLocalStorageSpec>
@end
Then update our implementation to use NSUserDefaults
with a custom suite name.
// RCTNativeLocalStorage.m
// TurboModuleExample
#import "RCTNativeLocalStorage.h"
static NSString *const RCTNativeLocalStorageKey = @"local-storage";
@interface RCTNativeLocalStorage()
@property (strong, nonatomic) NSUserDefaults *localStorage;
@end
@implementation RCTNativeLocalStorage
- (id) init {
if (self = [super init]) {
_localStorage = [[NSUserDefaults alloc] initWithSuiteName:RCTNativeLocalStorageKey];
}
return self;
}
- (std::shared_ptr<facebook::react::TurboModule>)getTurboModule:(const facebook::react::ObjCTurboModule::InitParams &)params {
return std::make_shared<facebook::react::NativeLocalStorageSpecJSI>(params);
}
- (NSString * _Nullable)getItem:(NSString *)key {
return [self.localStorage stringForKey:key];
}
- (void)setItem:(NSString *)value
key:(NSString *)key {
[self.localStorage setObject:value forKey:key];
}
- (void)removeItem:(NSString *)key {
[self.localStorage removeObjectForKey:key];
}
- (void)clear {
NSDictionary *keys = [self.localStorage dictionaryRepresentation];
for (NSString *key in keys) {
[self removeItem:key];
}
}
+ (NSString *)moduleName
{
return @"NativeLocalStorage";
}
@end
Important things to note:
- You can use Xcode to jump to the Codegen
@protocol NativeLocalStorageSpec
. You can also use Xcode to generate stubs for you.
Register the Native Module in your app
The last step consist in updating the package.json
to tell React Native about the link between the JS specs of the Native Module and the concrete implementation of those specs in native code.
Modify the package.json
as it follows:
"start": "react-native start",
"test": "jest"
},
"codegenConfig": {
"name": "AppSpecs",
"type": "modules",
"jsSrcsDir": "specs",
"android": {
"javaPackageName": "com.sampleapp.specs"
}
"ios":
"modulesProvider": {
"NativeLocalStorage": "RCTNativeLocalStorage"
}
},
"dependencies": {
At this point, you need to re-install the pods to make sure that codegen runs again to generate the new files:
# from the ios folder
bundle exec pod install
open SampleApp.xcworkspace
If you now build your application from Xcode, you should be able to build successfully.
Build and run your code on a Simulator
- npm
- Yarn
npm run ios
yarn run ios